How to create a sliding sidebar

A sliding sidebar is a wonderful way to add extra navigation or content without taking up too much screen space. I'll teach you how to make a sliding sidebar with HTML and CSS in this short tutorial. With just a few lines of code, you can add this useful feature to your website and provide your visitors with a more streamlined and pleasant browsing experience.
I'll go over the following subjects in this tutorial:
- Creating the HTML layout for the main content section and sidebar
- CSS styling of the sidebar and main content section
- When a button is clicked, JavaScript code is added to allow sliding animation.
Preview
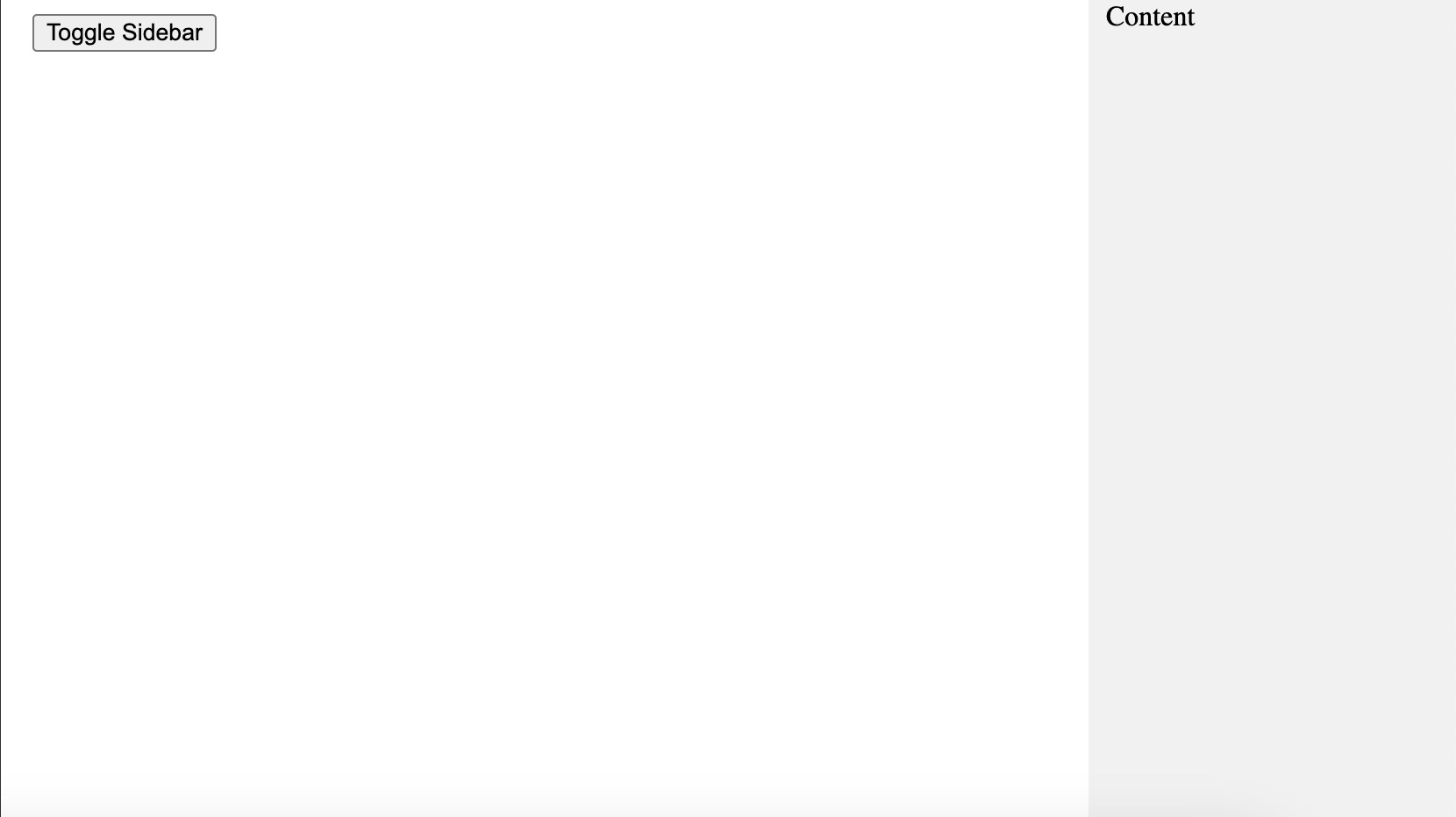
HTML Code
<!DOCTYPE html>
<html>
<head>
<title>Sliding Sidebar Example</title>
</head>
<body>
<div class="sidebar">
<!-- Add content for the sidebar here -->
Content
</div>
<div class="main-content">
<!-- Add content for the main content area here -->
<button class="toggle-button">Toggle Sidebar</button>
</div>
</body>
</html>
CSS Code
.sidebar {
position: fixed;
top: 0;
right: -200px;
width: 200px;
height: 100%;
background-color: #f1f1f1;
transition: 0.5s;
padding-left: 10px;
}
.show {
right: 0;
}
.main-content {
margin-left: 10px;
height: 100%;
background-color: #fff;
}
JavaScript Code
const sidebar = document.querySelector(".sidebar");
const toggleButton = document.querySelector(".toggle-button");
toggleButton.addEventListener("click", () => {
sidebar.classList.toggle("show");
});
How does it work?
The trick here is that we set the "sidebar" class with a fixed position and a width of 200px. We also set the position on the x-axis to -200px, which makes the sidebar invisible. Essentially, we move the sidebar out of the view.
When we click the button we activate the ".show" class that will set the "right" property to zero. This will move the element into the viewable area of the screen.
The "transition: 0.5s" property is used for the transition effect.